Today I want to discuss the how’s and why’s of a Backbone.JS (http://backbonejs.org/) implementation.
Ultimately, doing client side MVC, you come to face to face with Google’s popular Angular framework. Many people will question you if you use anything but Angular. Another architect I met summed it up well though. He said that if you have to teach a team Angular, it takes some time, because you have to learn the “Angular” way of doing things. using Backbone, it’s straight Model View Controller setup. There’s really nothing new in there to learn, and I’m a big fan of eliminating learning curves for teams when possible.
So, that’s as good a reason as any. Now, once you decided to go down the Backbone road, there’s a lot of opinionated decisions you have to make. Ironic, since Backbone is supposed to be “opinionated” according to some definitions. Personally I think there were still too many choices left up to the developer… ergo pitfalls.
Folder Structure
The first thing you have to decide is how you want to setup your project.
If you’re smart, you want to do something that will make sense to programmers that come later.
Here is what we ended up using:
[public] |-- [scss] |-- [img] |-- [vendor] (where you stuff 3rd party plugins) |-- [js] |-- [lib] (external scripts: handlebars, foundations, i18next, etc) |-- [collections] (your backbone extended collections) |-- [models] (your backbone extended models) |-- [templates] (handlebars templates for programmatic views) |-- [views] (your backbone extended views) |-- app.js (configure your backbone app) |-- main.js |-- require-config.js (setup require.js dependencies) |-- router.js (setup your routes) |-- [locales] |-- [tests] |-- Gruntfile.js (builds the project) |-- package.json (contains all the packages...) |-- server.js (contains the Node.js website configuration) |-- build.js (optimizes javascript files) |-- 404.html |-- favicon.ico |-- index.html
This came from Mathew LeBlanc’s excellent Backbone.js course on Lynda.com. Six months ago, I tried to follow Addy Osmani’s Backbone book, only to find it’s source code was out of date and didn’t work. There are some good ideas in it, but, the code’s out of date.
Here’s a short description of the 3rd party scripts we started with:
- Backbone – http://backbonejs.org/ The MV* framework scripts
- Foundation – http://foundation.zurb.com/ The front-end framework. I know it’s not Twitter Bootstrap…
- Handlebars – http://handlebarsjs.com/ Template engine. Backbone is bundled with Underscore, but it is limited for large projects.
- i18next.amd – http://i18next.com/ Internationalization. Simple, flexible. Require.js necessitates we use the AMD version of this library.
- jQuery – http://jquery.com/
- RequireJS – http://requirejs.org/ Load modules on demand. Improves speed… but it took a while to get just right.
Style Sheets
Foundation handles the mobile-first design.
For our own CSS organization, we follow the SMACSS (Scalable and Modular Architecture for CSS – http://smacss.com/) approach. On top of that, we’re using SASS with Compass which generates the .scss files.
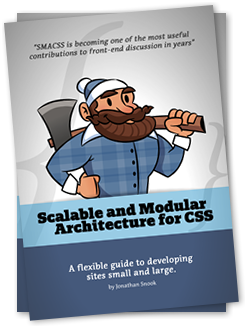
Scalable and Modular Architecture for CSS
SASS and LESS are popular CSS authoring frameworks (Foundation is built on SASS, so, it aligns better than LESS for us). That means they manage and compile CSS code for your project. To get the build/compile functionality, we’re using Compass, which is written in Ruby, so you need Ruby installed for it to work.
The folder structure ends up looking like this:
[public] |-- [css] |-- main.css (generated) |-- [scss] |-- [base] |-- [layout] |-- [module] |-- [state] |-- [theme] |-- _mixins.scss |-- _variables.scss |-- main.scss
Now let’s explain that a bit.
The base structure comes from SMACSS.
main.scss: this is automatically generated via imports from other scss files. This is the compilation process, and thus the only CSS file that will be minified.
_mixins.scss: Custom mixins.
_variables.scss: Contains scss variables (colors/fonts).
You define styles in base, layout, module, state, and theme.
So if you create a Backbone View called “medical-survey.js” then the handlebars template would be “medical-survey.html” and the style sheet would be “medical-survey.scss”
On small projects, you might think that’s annoying, but on a large scale project, this is very handy. It allows us to chunk out work, while still compiling it all into a single, minified styles sheet at the end.
The important part of SASS comes with this setup line of code:
sass --watch scss:css --style compressed
This tells the SASS (running via Ruby/Compass) to watch the folders under scss. SASS will find all the .scss files and import them into a single main.scss file. This file will be compiled into the final main.css. This file *will* come out very large. Even with the white space stripped out, it will often be huge and can slow down your site unacceptably…
Minification and Compression
So, if you implemented Require.js in the project to handle the efficient loading of Javascript files. What do you do about the massive CSS file you just generated using SASS above? If you are using Node.js, there’s a solution in the middleware. Compression.
The website discussing it is here:
https://github.com/expressjs/compression
This will use gzip to decrease the file size when loading the website.
There’s a lot of thought, effort, and detail complete missing from this blog. But if you have any questions, please let me know. My goal is always to help teams use simple and tested methods, but when we use newer techniques, we’re faced with not being able to get the best information about using it. This is definitely a major caveat to using technology developed in the last 3-5 years. We often do it anyway because new techniques are usually developed to resolve an annoyance we’ve been dealing with. Node.js resolves the annoyance of web servers like IIS and Apache being a bit slower and complex than we would like. It also adapts well in scalable scenarios.
Likewise, I don’t see any reasonable way to avoid the modern problem of having ridiculous amounts of Javascript and CSS. It’s just the modern problem. We don’t want to take the time hit to a project (and should not) to reinvent the wheel by rewriting things other developers have done via JQuery and other handy tools.
I have been coding CSS since 1998 and what began as a tool to *simplify* applying global (and thus branded) styles has grown into a monster; albeit a highly useful one, the most developers simply won’t take the time to really understand. SASS and LESS are simply ways for these developers to deal with the massive amount of CSS to compile it down into at least one file. And Compression makes it manageable. But it doesn’t solve the root problem:
The CSS cat has gotten out of the bag and no one is quite sure hot to get it back in.
The other root problem is unsolvable: the companies building the browsers will never follow a standard unless it’s the “standard” they made. Just accept it and you’ll be happier.
This is really an impressive post and seems clear.